Customize font from layout for TextView
In android layout textview
with font-family option with three fonts are given by default.If we have to add our own custom fonts from assert we have to do it by programmatically by adding these lines of code :
TextView name = (TextView)findViewById(R.id.name);
Typeface type=Typeface.createFromAsset(getAssets(),"fonts/RobotoSlabRegular.ttf");
txtyour.setTypeface(type);
Android 8.0 (API level 26) introduces a new feature, Fonts in XML, which lets you use fonts as resources. You can add the
font
file in the res/font/
folder to bundle fonts as resources. These fonts are compiled in your R
file and are automatically available in Android Studio. You can access the font resources with the help of a new resource type, font
. For example, to access a font resource, use @font/myfont
, or R.font.myfont
.To use the Fonts in XML feature on devices running Android 4.1 (API level 16) and higher, use the Support Library 26.
Add your font files in the font folder.
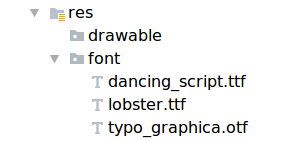
Creating a font family file in font folder
<?xml version="1.0" encoding="utf-8"?> <font-family xmlns:android="http://schemas.android.com/apk/res/android"> <font android:fontStyle="normal" android:fontWeight="400" android:font="@font/lobster_regular" /> <font android:fontStyle="italic" android:fontWeight="400" android:font="@font/lobster_italic" /> </font-family>
In the layout XML file, set the
fontFamily
attribute to the font file you want to access. <TextView
android:layout_width="wrap_content"
android:layout_height="wrap_content"
android:fontFamily="@font/lobster"/>
Adding fonts to style
<style name="customfontstyle" parent="@android:style/TextAppearance.Small"> <item name="android:fontFamily">@font/lobster</item> </style>
Using fonts programmatically
Typeface typeface = getResources().getFont(R.font.myfont); textView.setTypeface(typeface);
Below code Old way
Like this there are different ways to add customized fonts to textview in android. we will today learn the easiest way to do this.
Create a FontTextView.class:
@SuppressLint("AppCompatCustomView") public class FontTextView extends TextView { public FontTextView(Context context) { super(context); init(null, 0); } public FontTextView(Context context, @Nullable AttributeSet attrs) { super(context, attrs); init(attrs, 0); } public FontTextView(Context context, @Nullable AttributeSet attrs, int defStyleAttr) { super(context, attrs, defStyleAttr); init(attrs, defStyleAttr); } @RequiresApi(api = Build.VERSION_CODES.LOLLIPOP) public FontTextView(Context context, @Nullable AttributeSet attrs, int defStyleAttr, int defStyleRes) { super(context, attrs, defStyleAttr, defStyleRes); init(attrs, defStyleAttr); } private void init(AttributeSet attrs, int defStyleAttr) { TypedArray attrArray = getContext().obtainStyledAttributes(attrs,R.styleable.MyTextView, defStyleAttr, 0); initAttributes(attrArray); } protected void initAttributes(TypedArray attrArray) { String textStyle = attrArray.getString(R.styleable.MyTextView_myTextStyle); if (textStyle == null || textStyle.equals(null) || textStyle.equals("")) { //do nothing it will override default typeface from android } else { Typeface tf = Typeface.createFromAsset(getContext().getAssets(),textStyle); setTypeface(tf); } } }
Create attrs.xml in values resource folder:
<?xml version="1.0" encoding="utf-8"?> <resources> <declare-styleable name="MyTextView"> <attr name="myTextStyle" format="string"></attr> </declare-styleable> </resources>
In strings.xml in values resource folder:
<string name="robo_slab_regular">"font/RobotoSlabRegular.ttf"</string>
Now its time to use the FontTextView in layout:
<com.example.FontTextView android:id="@+id/tvName" android:layout_width="wrap_content" android:layout_height="wrap_content" android:text="PM" android:textColor="@android:color/white" app:myTextStyle="@string/robo_slab_regular" />
Comments
Post a Comment